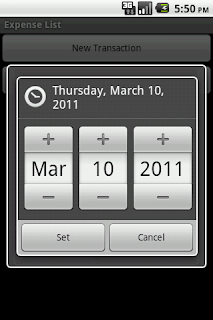
If your edit text is etDialogDate,
etDialogDate.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
String dateText = ((TextView)v).getText().toString();
int year,month,day;
String temp[]=dateText.split("-");
year = Integer.valueOf(temp[0]);
month = Integer.valueOf(temp[1]);
day= Integer.valueOf(temp[2]);
DatePickerDialog dtpkrdlg = new
@Override
public void onClick(View v) {
String dateText = ((TextView)v).getText().toString();
int year,month,day;
String temp[]=dateText.split("-");
year = Integer.valueOf(temp[0]);
month = Integer.valueOf(temp[1]);
day= Integer.valueOf(temp[2]);
DatePickerDialog dtpkrdlg = new
DatePickerDialog(ExpenseList.this, 0,
new DateSetListener(), year, month-1, day);
dtpkrdlg.show();
}});
dtpkrdlg.show();
}});
Now this will show the datepicker dialog with initial value as date shown in edittext.
Once the date is set in the dialog it will call the callback function using DateSetListener. Let us define this DateSetListener class as follows
class DateSetListener implements DatePickerDialog.OnDateSetListener{
@Override
public void onDateSet(DatePicker view,
@Override
public void onDateSet(DatePicker view,
int year, int monthOfYear,
int dayOfMonth) {
etDialogDate.setText(""+year+"-"+
int dayOfMonth) {
etDialogDate.setText(""+year+"-"+
(monthOfYear+1)+"-"+dayOfMonth);
}
}
}
}
Now any date set in the dialog is displayed back in our edittext widget.
Observe that we have used monthOfYear+1 because in Android Jan is 0 not 1.
No comments:
Post a Comment